Archive Page 4
October 20th, 2014 by admin
Newry-based financial software firm First Derivatives has acquired a majority shareholding in big data analytics company Kx Systems for £36m (€44m).
KX has historically had a hard time penetrating markets outside finance, FD have a good sales team and previously acquired a marketing company in Philadelphia, hopefully this is the chance for kdb+ to go mainstream.
However it’s a worry that FD (First Derivatives) may increasingly “encourage” purchasing of the delta platform bundle rather than stand-alone kdb+. With the smaller margins outside of finance, will FD take a risk and open up the database. (Would FD have been a supporter of the 32-bit version becoming free for commercial use?) There’s a large number of individuals in off-shore locations that want to learn kdb+, FD could be incentivized to discourage that as it would hurt their consulting business.
It’s also interesting to consider companies that have already invested in KX technology and whether they will continue to do so
- Competing consulting firms that specialise in kdb+ won’t take this as good news.
- Panopticon/Datawatch based their visualization system on kdb+ (OEM license), they probably regret that now, given that their visualization software directly competes with FD’s dashboards.
- Companies that had used kdb+ as part of their trading platform stack may consider FD a competitor as they also offer a trading platform.
What do you think? Will this lead to wider adoption? a growing platform?
October 8th, 2014 by admin
kdb+ London Contract – £800 p/day
kdb+ belfast Citigroup Contract £400 p/day
Java Poland £150 p/day
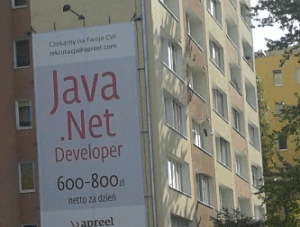
September 8th, 2014 by admin
sqlDashboards are included as a bundle with qStudio, part of that package is a command line utility called sqlChart that allows generating customized sql charts from the command line.
Checkout the video to see how you can create a chart based on data from a kdb+ database in 2 minutes:
The sqlChart page has all the documentation you need, Download the qstudio.zip to try it now.
The q Code
Help Screen
September 8th, 2014 by admin
I always like to investigate new technology and this week I found a nice automatic technique for improved cache use that I had previously seen some people manually write.
Consider a database query with three steps (three SQL SELECTs), some databases may pass results of each step to temporary tables in main memory. When the first step is finished, these intermediate results are passed back into CPU cache to be transformed by the second step, then back into a new temporary table in main memory, and so on.
To eliminate this back-and-forth, vector-based statistical functions can be pipelined, with the output of one function becoming input for the next, whose output feeds a third function, etc. Intermediate results stay in the pipeline inside CPU cache, with only the full result being materialized at the end.
This technology is part of ExtremeDB, they have a video that explains it well:
Moving Averages Stock Price Example
This is what the actual code would look like to calculate the 5-day and 21-day moving averages for a stock and detect the points where the faster moving average (5-day) crosses over or under the slower moving average (21-day):
- Two invocations of ‘seq_window_agg_avg’ execute over the closing price sequence, ‘ClosePrice’, to obtain 5-day and 21-day moving averages.
- The function ‘seq_sub’ subtracts 21- from 5-day moving averages;
- The result “feeds” a fourth function, ‘seq_cross’, to identify where the 5- and 21-day moving averages cross.
- Finally, the function ‘seq_map’ maps the crossovers to the original ‘ClosePrice’ sequence, returning closing prices where the moving averages crossed.
This approach eliminates the need to create, populate and query temporary tables outside CPU cache in main memory. One “tile” of input data is processed by each invocation of ‘mco_seq_window_agg_avg_double()’, each time producing one tile of output that is passed to ‘mco_seq_sub_double()’ which, in turn, produces one tile of output that is passed as input to mco_seq_cross_double(), which produces one tile of output that is passed to mco_seq_map_double(). When the last function, mco_seq_map_double() has exhausted its input, the whole process repeats from the top to produce new tiles for additional processing.
A very cool idea!
And yes, ExtremeDB are the same guys that posted the top Stac M3 benchmark for a while (in 2012/13 I think).
April 10th, 2014 by admin
Kdb does not have built-in functions for bitwise and,or,xor operations, we are going to create a C DLL extension that provides bitwise operators.
To download the source and definitions for and/or/xor bitwise operations click here
If your new to extending Kdb+ see this tutorial on writing a DLL for Kdb+
I also thought this would be a good opportunity to look at using q-code to generate C code, to generate Kdb Dll’s. When converting an existing library to work with Kdb or writing similar functions for different types, there’s a lot of repetitive coding. Either you can use macros or you can use one language to generate another, to save a lot of typing. Let’s look at bitwise operations as an example of what I mean:
If I wanted to write a bitwise and function band that takes two lists and performs a corresponding operation, we could write the C functions like so:
Notice the similarity between the bandJJ and bandII functions. Plus if we want to handle the case where the second argument is an atom we need an entire other set of C functions similar to bandJ to handle that. This is soon beginning to spiral beyond an easy copy paste job. Instead I used the following 20 lines of q code to generate the 130 lines of C code:
This generates my C code, a .def file exporting the functions that I want to provide and creates a .q file that loads those functions into kdb. Which means any time I add a function or want to support a new type, everything is done for me. let’s look at our functions in action:
Yes the q code is messy (it could be improved) but the concept of dynamically generating your imports can be a real time saver.
This code is not intended for reliability or performance, use at your own risk! If however bitwise operations are a topic that interest you I recommend this article on: Writing a fast vectorised OR function for Kdb.
March 30th, 2014 by admin
While doing the project euler programming challenges it annoyed me how verbose the java answers would have to be compared to kdb. Then I got to wondering if I could create functions like til,mod,where,asc etc. in java and use them to create really short answers. Once I had the basic functions working, I wondered if I could get a working q) prompt…
I ended up with a prompt that let me run the following valid q code within the java runtime:
where[or[ =[0; mod[til[1000];3]]; =[0; mod[til[1000];5]] ]]
Download the jkdb functional kdb code
The Code
How it Works
My Jkdb class has a number of statically defined functions that accept arrays of ints/doubles and return arrays/atoms of int/double. An example is asc:
In the background each line you type has the square brackets and semi-colons converted to () and comma which are now valid java function calls. The amended string gets added to a .java file, compiled, ran and the output shown each time you press enter. Since I statically imported my class that has the til(x),max(x) etc functions, the code is valid java and works. This is why we can only use functional[x;y] form calls and not the infix notation x func y as I didn’t want to write a parser.
Interesting Points that would occur in Kdb
- All of the functions usually accept int,double, int[],double[] and boolean[] as their arguments and the various combinations. It is annoying having to copy paste these various combinations, I assume in kdb they use C macros to generate the functions, we could do something similar by writing java code to write the java functions.
- The functions I wrote often modify the array passed in, this gives us insight to why in the kdb C api you have to reference count properly, when the reference is 0 the structure is probably reused.
What use is this?
Almost entirely useless 🙂 I use the java class to occasionally spit out some sample data for tests I write. It was however insightful to get an idea of how kdb works under the covers. If you found this interesting you might also like kona an open source implementation of kdb.
I’d be interested in hearing:
- Have you tried kona? Did it work well?
- Have you found a functional library that gives you similar expressiveness to kdb for java?
- Tried Scala? Clojure?
The Jkdb provided functions are:
til where |
equal= |
index@ |
choose(x,y) ? – only supports x and y as int’s to choose random numbers |
|
or(x,y) and(x,y) |
mul(x,y) add(x,y) |
mod(x,y) |
|
floor ceiling abs |
cos sin tan |
acos asin atan |
sqrt |
|
asc desc max min reverse |
sum sums prd prds |
|
set(String key, Object value) |
get(String key) |
June 24th, 2013 by admin
http://www.kx.com/press-releases-130610.php
Palo Alto (10 Jun 2013) – Kx Systems, the leader in high-performance database and time-series analysis, has announced today the release of kdb+ v3.1 as well as its latest benchmark results for the STAC (Securities Technology Analysis Center) summit in Chicago. The results of the STAC M3™ vendor-independent market data benchmark suite demonstrate very significant performance improvements in v3.1, with results up to 8x faster than any previous STAC M3 benchmarks.
STAC ReportThe tests highlighted Kx’s unparalleled performance in such real-world calculations as generating the complete NBBO (National Best Bid and Offer) for one day, on thousands of symbols. Compared to previous best results, v3.1 runs over 4x faster, taking about half a minute to read, calculate, and write the entire table. In other benchmarks, calculations such as VWAPs (Volume Weighted Average Prices) on tick data were more than 8x faster….
It’s great to see kx continue to make speed improvements.
May 26th, 2013 by admin
Andrey Zholos announced a new version of q Math Library:
qml 0.4 is out:
http://althenia.net/qml
Now compatible with kdb+ 3.0 and whatever implementation of LAPACK your
distro provides.
Thanks to everyone who prompted me to continue working on this, and
especially to Jim Schmitz for his invaluable ideas, Kim Kuen Tang
for providing an interim version, and Kx for making a binary-
incompatible change to the interface
If there’s a permissively-licensed numerical library you’d like to see
included in the next version, let Andrey know. Or just submit a patch.
April 2nd, 2013 by admin
The excellent Q For Mortals: A Tutorial In Q Programming by Jeffry Borror will soon be updated to version 3.
Jeff mentioned it at the recent NY user meeting.
You can read q for mortals online for free at: http://code.kx.com/wiki/JB:QforMortals2/contents
version 2 – added a new chapter on kdb database disk storage.
He said to get in touch if there’s anything you feel you would really like added.
UPDATE December 2015
q for mortals 3 is now out.
Q for Mortals Version 3 is a thorough presentation of the q programming language and an introduction to the kdb+ database. It is a complete rewrite of the original Q for Mortals that is current with q3.3. The presentation is derived from classes taught by the author at international financial institutions over the last decade. It is a series of tutorials based on q snippets intended to be entered interactively into the q console by the reader. The text takes its subject seriously but not itself. Technical explanations are augmented by mathematical observations, references to general programming concepts and other programming languages, and bad jokes. Coding style recommendations and advice to avoid gotchas appear liberally throughout. Examples are as simple as they can be but no simpler.
- Chapter 1, Q Shock and Awe, provides a piquant panorama of the power of q and its dazzling zen-like nature.
- Chapter 2 describes the base data types of q.
- Chapter 3 discusses lists, the fundamental data structure of q
- Chapter 4 presents the basic operators.
- Chapter 5 introduces dictionaries, which associate keys and values.
- Chapter 6 presents an in-depth description of functions and q’s constructs for functional programming.
- Chapter 7 demonstrates transforming data from one type to another.
- Chapter 8 introduces tables and keyed tables, the fundamental data structures for kdb.
- Chapter 9 describes q-sql and all the methods to manipulate tables.
- Chapter 10 presents ways to control execution of q programs.
- Chapter 11 covers file and interprocess communication I/O
- Chapter 12 describes workspace organization and management.
- Chapter 13 discusses system commands and command line parameters.
- Chapter 14 serves as an introduction to the kdb+ database. M
Available at all good bookstores.
http://www.amazon.com/For-Mortals-Version-Introduction-Programming/dp/0692573674
qTips is also good: http://www.amazon.co.uk/Tips-Fast-Scalable-Maintainable-Kdb/dp/9881389909
April 2nd, 2013 by admin
Added four new tutorials for those starting to learn kdb: