Archive for the 'kdb+' Category
March 30th, 2014 by admin
While doing the project euler programming challenges it annoyed me how verbose the java answers would have to be compared to kdb. Then I got to wondering if I could create functions like til,mod,where,asc etc. in java and use them to create really short answers. Once I had the basic functions working, I wondered if I could get a working q) prompt…
I ended up with a prompt that let me run the following valid q code within the java runtime:
where[or[ =[0; mod[til[1000];3]]; =[0; mod[til[1000];5]] ]]
Download the jkdb functional kdb code
The Code
How it Works
My Jkdb class has a number of statically defined functions that accept arrays of ints/doubles and return arrays/atoms of int/double. An example is asc:
In the background each line you type has the square brackets and semi-colons converted to () and comma which are now valid java function calls. The amended string gets added to a .java file, compiled, ran and the output shown each time you press enter. Since I statically imported my class that has the til(x),max(x) etc functions, the code is valid java and works. This is why we can only use functional[x;y] form calls and not the infix notation x func y as I didn’t want to write a parser.
Interesting Points that would occur in Kdb
- All of the functions usually accept int,double, int[],double[] and boolean[] as their arguments and the various combinations. It is annoying having to copy paste these various combinations, I assume in kdb they use C macros to generate the functions, we could do something similar by writing java code to write the java functions.
- The functions I wrote often modify the array passed in, this gives us insight to why in the kdb C api you have to reference count properly, when the reference is 0 the structure is probably reused.
What use is this?
Almost entirely useless 🙂 I use the java class to occasionally spit out some sample data for tests I write. It was however insightful to get an idea of how kdb works under the covers. If you found this interesting you might also like kona an open source implementation of kdb.
I’d be interested in hearing:
- Have you tried kona? Did it work well?
- Have you found a functional library that gives you similar expressiveness to kdb for java?
- Tried Scala? Clojure?
The Jkdb provided functions are:
til where |
equal= |
index@ |
choose(x,y) ? – only supports x and y as int’s to choose random numbers |
|
or(x,y) and(x,y) |
mul(x,y) add(x,y) |
mod(x,y) |
|
floor ceiling abs |
cos sin tan |
acos asin atan |
sqrt |
|
asc desc max min reverse |
sum sums prd prds |
|
set(String key, Object value) |
get(String key) |
March 29th, 2014 by Ryan Hamilton
Let’s look at how to write moving average analytics in q for the kdb database. As example data (mcd.csv) we are going to use stock price data for McDonalds MCD. The below code will download historical stock data for MCD and place it into table t:
Simple Moving Average
The simple moving average can be used to smooth out fluctuating data to identify overall trends and cycles. The simple moving average is the mean of the data points and weights every value in the calculation equally. For example to find the moving average price of a stock for the past ten days, we simply add the daily price for those ten days and divide by ten. This window of size ten days then moves across the dates, using the values within the window to find the average. Here’s the code in kdb for 10/20 day moving average and the resultant chart.
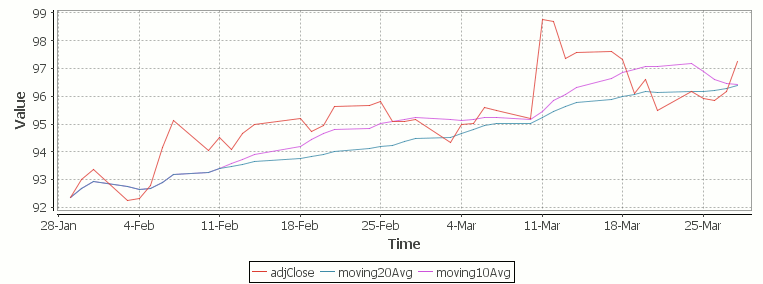
Simple Moving Average Stock Chart Kdb (Produced using qStudio)
What Exponential Moving Average is and how to calculate it
One of the issues with the simple moving average is that it gives every day an equal weighting. For many purposes it makes more sense to give the more recent days a higher weighting, one method of doing this is by using the Exponential Moving Average. This uses an exponentially decreasing weight for dates further in the past.The simplest form of exponential smoothing is given by the formula:

where α is the smoothing factor, and 0 < α < 1. In other words, the smoothed statistic st is a simple weighted average of the previous observation xt-1 and the previous smoothed statistic st−1.
This table displays how the various weights/EMAs are calculated given the values 1,2,3,4,8,10,20 and a smoothing factor of 0.7: (excel spreadsheet)
Values |
EMA |
|
Power |
Weight |
Power*Weight |
|
EMA (text using previous value) |
1 |
1 |
|
6 |
0.0005103 |
0.0005103 |
|
1 |
2 |
1.7 |
|
5 |
0.001701 |
0.003402 |
|
(0.7*2)+(0.3*1) |
3 |
2.61 |
|
4 |
0.00567 |
0.01701 |
|
(0.7*3)+(0.3*1.7) |
4 |
3.583 |
|
3 |
0.0189 |
0.0756 |
|
(0.7*4)+(0.3*2.61) |
8 |
6.6749 |
|
2 |
0.063 |
0.504 |
|
(0.7*8)+(0.3*3.583) |
10 |
9.00247 |
|
1 |
0.21 |
2.1 |
|
(0.7*10)+(0.3*6.6749) |
20 |
16.700741 |
|
0 |
0.7 |
14 |
|
(0.7*20)+(0.3*9.00247) |
To perform this calculation in kdb we can do the following:
(This code was originally posted to the google mail list by Attila, the full discussion can be found here)
This backslash adverb works as
The alternate syntax generalizes to functions of 3 or more arguments where the first argument is used as the initial value and the arguments are corresponding elements from the lists:
Exponential Moving Average Chart
Finally we take our formula and apply it to our stock pricing data, allowing us to see the exponential moving average for two different smoothing factors:
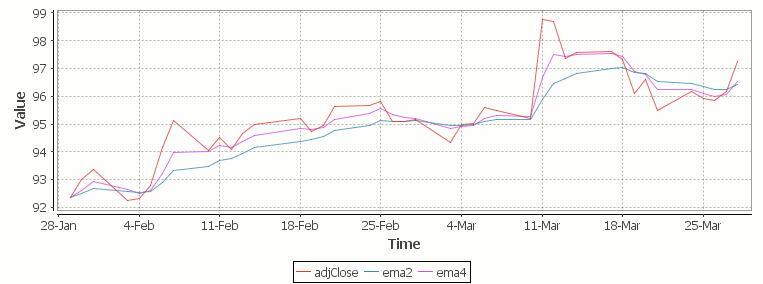
Exponential Moving Average Stock Price Chart produced using qStudio
As you can see with EMA we can prioritize more recent values using a chosen smoothing factor to decide the balance between recent and historical data.
Writing kdb analytics such as Exponential Moving Average is covered in our kdb training course, we regularly provide training courses in London, New York, Asia or our online kdb course is available to start right now.
March 4th, 2014 by John Dempster
qStudio is an IDE for kdb+, you can download it now.
The latest 1.31 release introduces a number of nice interface additions as requested by users:
Command Palette
Quick change server -> Hit Ctrl+P to try the new Command Palette
It lets you run common qStudio actions by fuzzy matching on keywords:

Jump to Definition
Hit Ctrl+U Ctrl+I to get an outline of the current file.
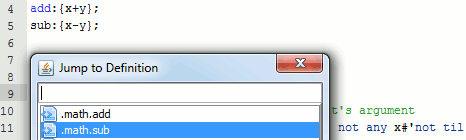
Or press Ctrl+D on a function call to jump to where it is defined.
Added User Preferences
Users had mentioned a number of connection and interface issues they wanted to be able to customize, these have been added:
– Customize the editor font and it’s background color (per server setting, useful for red warning color when connected to prod machines)
– keep same connection open for every query
– Wrap every query with selected text before and after
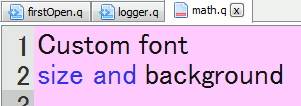
Eval Line by Line
Do you ever query a line, move down, query next line, move down ….
Well try control+shift+enter within the code editor.
It evaluates the current line, returns it’s value and shifts to the next line.
e.g. for the code:
a:11
b:a*rand[10]
c:b*a
The console would show:
q)a:11
11
q)b:a*rand[10]
55
q)c:b*a
605
The qStudio help guide contains more details of all functionality.
December 15th, 2013 by Ryan Hamilton
In a previous post I looked at using the monte carlo method in kdb to find the outcome of rolling two dice. I also posed the question:
How can we find the value of Pi using the Monte Carlo method?
If we randomly chose an (x,y) location, we could calculate the distance of that points location ( sqrt[x²+y²] ) from the origin, this would tell us if that point lay within a circle or outside a circle, by whether the length was greater than our radius. The ratio of the points that fall within the circle relative to the square that bounds our circle, gives us the ratio of the areas.
A diagram is probably the easiest way to visualize this problem. If we generate two lists of random numbers between -1 to 1. And use those as the coordinates (x,y), then create a scatter plot that plots (x,y) in one colour where x²+y² is greater than 1 and a different colour where it is less than 1, we would find a pattern emerging…in qStudio it looks like this:
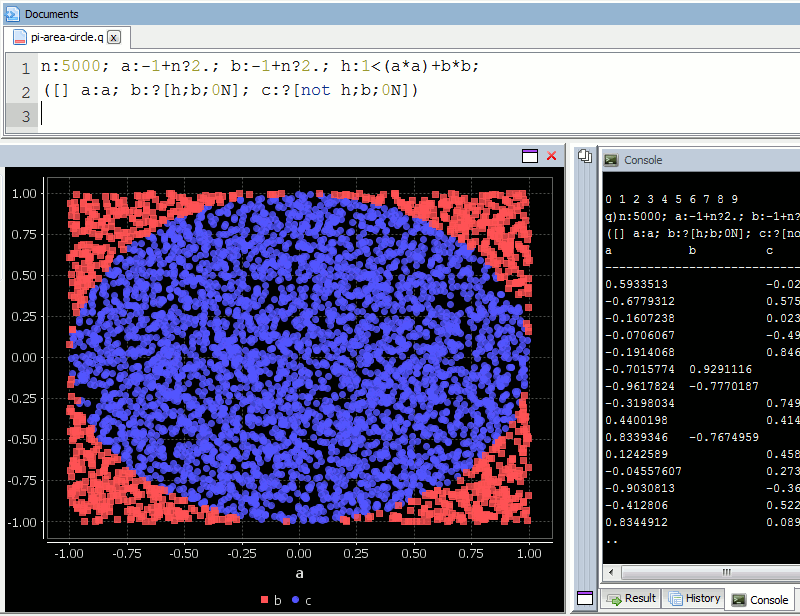
We know the area of our bounding square is 2*2 and that areaOfCircle=Pi*r*r, where r is 1. Therefore we can see that:
areaOfCircle = shadedRatio*areaOfSquare
Pi = shadedRatio * 4
q)4*sum[not h]%count h
3.141208
The wikipedia article on monte carlo method steps through this same example, as you can see their diagram looks similar:
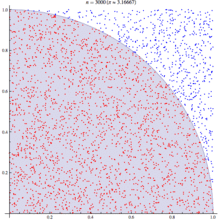
December 10th, 2013 by Ryan Hamilton
In a previous post I looked at using the monte carlo method in kdb to find the outcome of rolling two dice. I also posed the question:
How many people do you need before the odds are good (greater than 50%) that at least two of them share a birthday?
In our kdb+ training courses I always advise breaking the problem down step by step, in this case:
- Consider making a function to examine the case where there are N people.
- Generate lists of N random numbers between 0-365 representing their birthdays.
- Find lists that contain collisions.
- Find the number of collisions per possibilities examined.
- Apply our function for finding the probability for N people to a list for many possibilities of N.
In kdb/q:
Plotting our data in using qStudio charting for kdb we get:
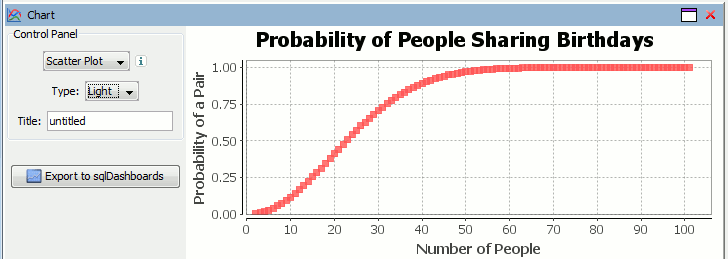
Therefore as you can see from either the q code or the graph, you need 23 people to ensure there’s a 50% chance that atleast 2 people in the room share a birthday. For more details see the wikipedia page. This still leaves us with the other problem of finding Pi using the monte carlo method in kdb.
December 3rd, 2013 by Ryan Hamilton
Performing probability questions in kdb/q is simple. I recently got asked how to find the probability of rolling a sum of 12 with two dice. We’ll look at two approaches to finding the likely outcomes in kdb/q:
Method 1 – Enumeration of all possibilities
Step by step we:
- Generate the possible outcomes for one die.
- Generate all permutations for possible outcomes of two dice, find the sum of the dice.
- Count the number of times each sum occurs and divide by all possible outcomes to get each probability.
Method 2 – Monte Carlo Simulation
Alternatively , if it hadn’t occurred to us to use cross to generate all possible outcomes, or for situations where there may be too many to consider. We can use Monte Carlo method to simulate random outcomes and then similarly group and count their probability.
q)1+900000?/:6 6 / random pairs of dice rolls
2 5 6 6 2 4 3 1 5 1 1 4 6 5 3 4 2 4 1 2 3 6 6 1 1 3 6 3 6 2 3 1 5 4 4 4 6 3 1 3 1 2 5..
4 4 4 5 1 4 3 6 5 1 4 4 3 4 1 1 1 5 4 5 2 4 4 4 5 2 1 2 6 5 2 4 3 1 4 2 1 1 3 4 6 5 1..
q)/ same as before, count frequency of each result
q)(2+til 11)#{x%sum x} count each group sum each flip 1+900000?/:6 6
2 | 0.02766
3 | 0.05559
4 | 0.08347
5 | 0.11085
6 | 0.1391822
7 | 0.1669078
8 | 0.1386156
9 | 0.1112589
10| 0.08317222
11| 0.05556222
12| 0.02773111
Therefore the probability of rolling a sum of 12 with two dice is 1/36 or 0.27777. Here’s the similar dice permutation problem performed in java.
Kdb Problem Questions
If you want to try using the monte carlo method yourself try answering these questions:
- The Birthday Paradox: How many people do you need before the odds are good (greater than 50%) that at least two of them share a birthday?
- Finding the value of Pi. Consider a square at the origin of a coordinate system with a side of length 1. Now consider a quarter circle inside of the square, this circle has a radius of 1, therefore its area is pi/4. For a point (X,Y) to be inside of a circle of radius 1, its distance from the origin (X ², Y²) will be less than or equal to 1. We can generate random (X,Y) positions and determine whether each of them are inside of the circle. The ratio of those inside to outside will give the area. (bonus points for using multiple threads)
November 12th, 2013 by John Dempster
qStudio is an IDE for kdb+ database by kx systems that allows querying kdb+ servers, charting results and browsing server objects from within the GUI.
Version 1.29 of qStudio is now available:
http://www.timestored.com/qstudio/
Changes in the latest version include a new dark theme for charts and the ability to run multiple instances of qStudio.
The changes were added as a few people had asked for a dark theme due to eye strain from staring at the white charts.
If you have any ideas for what you would like to see in the next version please let me know.
john AT timestored DOT com
Here’s a preview of a time series graph:
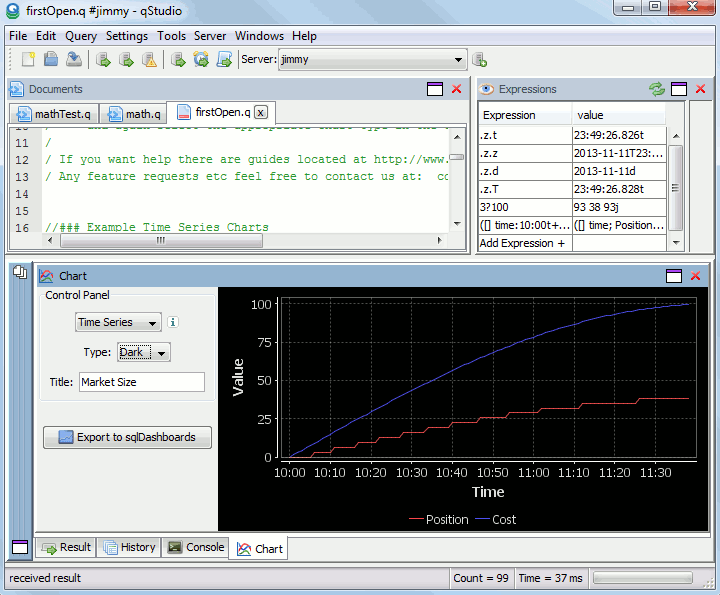
qStudio with a dark time series chart
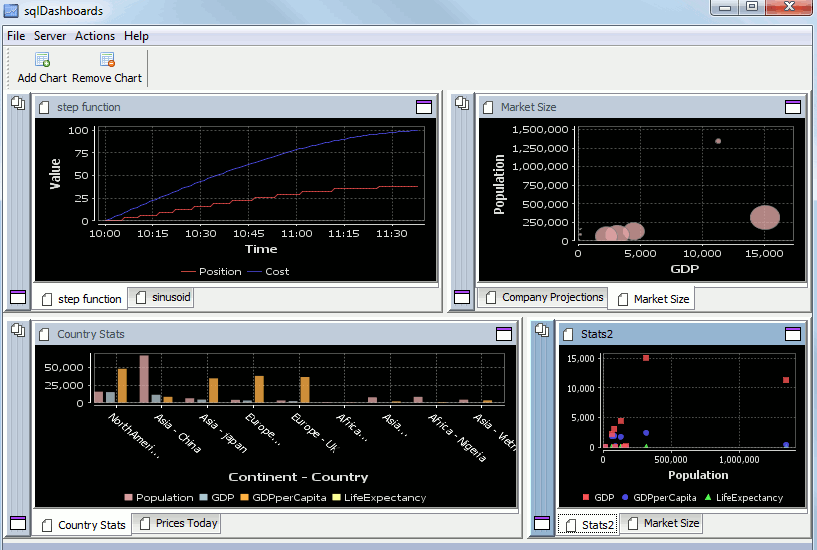
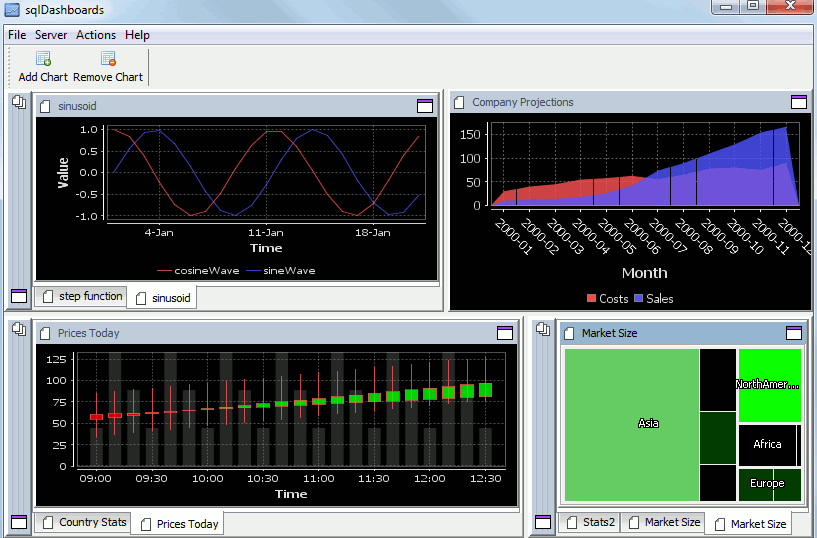
October 28th, 2013 by John Dempster
Typical feed handlers for kdb+ are for market data and trade data such as bloomberg B-Pipe or reuters market feeds. These feeds typically contain ticker symbol, bid price, ask price and the time. We’ve been working on something a little different, a twitter feed handler. With this feed handler you can subscribe to:
- A random sample of all tweets
- Certain search queries
- Locations, tweets for any trending queries will be downloaded for those areas
For each tweet we have associated meta data that includes: location, language, time of posting and number of favourites/retweets.
Now that we have our data in kdb+ we can analyse it like any other time-series data and look for interesting patterns. If you have worked on anything similar I would love to hear about it (john AT timestored.com). I find treating social media data as time-series data throws up many interesting possibilities, in future blog posts I’ll start digging into the data..
At TimeStored we have previously implemented a number of market data feed handlers. Handling reconnections, failover, data backfilling and data enrichment can be a tricky problem to get right, if you need a feed handler developed we provide kdb+ development, consulting and support services, please contact us.
Basic examples of Java kdb+ Feed handlers and C Feed Handlers are available on the site.
July 8th, 2013 by Ryan Hamilton
qStudio is an editor for kdb+ database by kx systems. Version 1.28 of qStudio is now available for download:
http://www.timestored.com/qstudio/
Changes in the latest version include:
- Added Csv Loader (pro)
- Added qUnit unit testing (pro)
- Bugfix to database management column copying.
- Export selection/table bugs fixed and launches excel (thanks Jeremy / Ken)
The Kdb+ Csv Loader allows loading local files onto a remote kdb+ server easily from within a GUI.
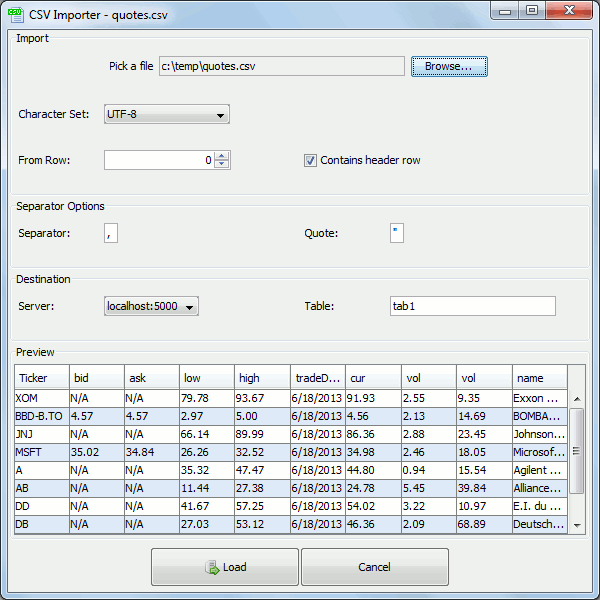
For step-by-step instructions see the qStudio loader help.
qUnit allows writing unit tests for q code in a format that will be familiar to all those who have used junit,cunit or a similar xunit based test framework. Tests depend on assertions and the results of a test run are shown as a table like so:
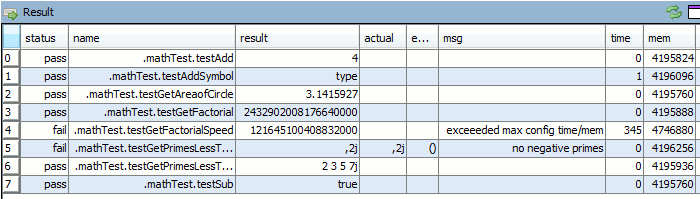
Tests are specified as functions that begin with the test** prefix and can have time or memory limits specified.
May 15th, 2013 by Ryan Hamilton
Sanket Agrawal just posted some very cool code to the k4 mailing list for finding the longest common sub-sequences. This actually solves a problem that some colleagues had where they wanted to combine two tickerplant feeds that never seemed to match up. Here’s an example:
q)/ assuming t is our perfect knowledge table
q)t
time sym size price
------------------------
09:00 A 8 7.343062
09:01 A 0 5.482385
09:02 B 5 8.847715
09:03 A 1 6.78881
09:04 B 5 3.432312
09:05 A 0 0.2801381
09:06 A 2 3.775222
09:07 B 3 1.676582
09:08 B 7 7.163578
09:09 B 4 3.300548
Let us now create two tables, u and v, neither of which contain all the data.
q)u:t except t 7 8
q)v:t except t 1 2 3 4
q)u
time sym size price
------------------------
09:00 A 8 7.343062
09:01 A 0 5.482385
09:02 B 5 8.847715
09:03 A 1 6.78881
09:04 B 5 3.432312
09:05 A 0 0.2801381
09:06 A 2 3.775222
09:09 B 4 3.300548
q)v
time sym size price
------------------------
09:00 A 8 7.343062
09:05 A 0 0.2801381
09:06 A 2 3.775222
09:07 B 3 1.676582
09:08 B 7 7.163578
09:09 B 4 3.300548
We can find the indices that differ using Sankets difftables function
q)p:diffTables[u;v;t `sym;`price`size]
q)show p 0; / rows in u that are not in v
1 2 3 4
q)show p 1; / rows in v that are not in u
3 4
/ combine together again
q)`time xasc (update src:`u from u),update src:`v from v p 1
time sym size price src
----------------------------
09:00 A 8 7.343062 u
09:01 A 0 5.482385 u
09:02 B 5 8.847715 u
09:03 A 1 6.78881 u
09:04 B 5 3.432312 u
09:05 A 0 0.2801381 u
09:06 A 2 3.775222 u
09:07 B 3 1.676582 v
09:08 B 7 7.163578 v
09:09 B 4 3.300548 u
q)t~`time xasc u,v p 1
1b
The code can be downloaded at:
http://code.kx.com/wsvn/code/contrib/sagrawal/lcs/miller.q
http://code.kx.com/wsvn/code/contrib/sagrawal/lcs/myers.q
Thanks Sanket.
Algorithm details:
Myers O(ND): http://www.xmailserver.org/diff2.pdf
Miller O(NP): http://www.bookoff.co.jp/files/ir_pr/6c/np_diff.pdf